How To Create a Conversational Voice Chatbot [No Code]
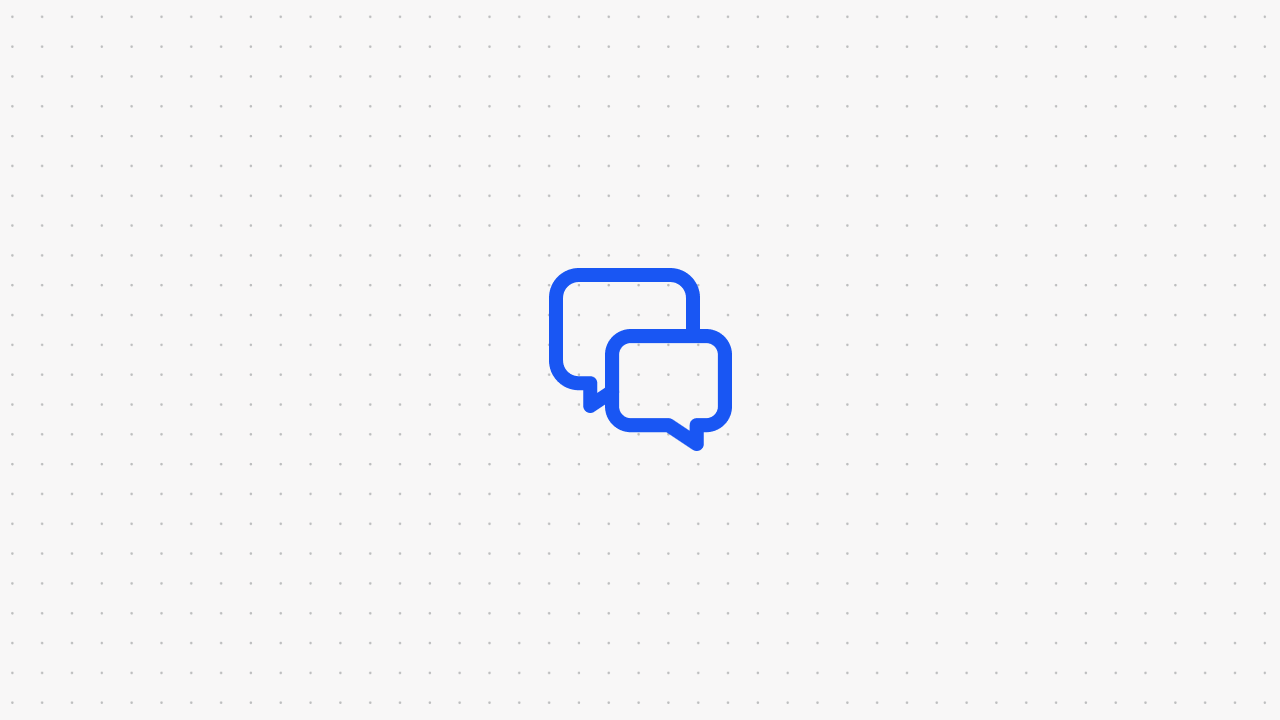
Apply for early access to new Voice AI features here.
Recently, OpenAI found itself in a bit of hot water after releasing a new AI voice for ChatGPT-4o that sounded strikingly similar to Scarlett Johansson’s character in “Her”. In the demo, the voice chatbot didn’t just converse—it also sang! The chatbot handled complex queries, provided detailed assistance, and told stories, all with a natural-sounding voice and the right emotional tone.
For businesses, this is a game-changer. Whether it’s a virtual assistant helping customers in a store, or a healthcare chatbot providing instant medical advice, the applications are vast. The benefits are clear: happier customers, lower costs, and a competitive edge.
Now is the perfect time for businesses to invest in voice chatbots, and there’s no better tool for creating these sophisticated AI-driven interfaces than Voiceflow. This article will show you how to create a voice chatbot, provide sample codes, and demonstrate how you can do it with Voiceflow in just 10 minutes.
What Is A Voice Chatbot? Definition
A voice chatbot is an AI-powered assistant that interacts with users through voice commands. Examples of voice chatbots include Siri, Alexa, Google Assistant, and ChatGPT-4o.
How Do AI-Powered Voice Chatbots Work?
AI-powered voice chatbots with a custom knowledge base (KB) works like this:
- Automatic Speech Recognition (ASR) converts spoken language into text. This involves the use of acoustic modeling, language modeling, and decoding algorithms to transcribe speech accurately.
- Natural Language Processing (NLP) interprets the transcribed text. This step involves tokenization, part-of-speech tagging, named entity recognition, and dependency parsing to understand user intent and extract relevant entities.
- The chatbot queries its custom Knowledge Base (KB) using semantic search and Retrieval Augmented Generation (RAG). Semantic search improves the retrieval of contextually relevant information, while RAG combines KB data with generative models to provide accurate and comprehensive responses.
- Intent Recognition and Response Generation employ large language models (LLMs) like BERT or GPT. These models match user intent with the appropriate response, leveraging data from the KB.
- Dialog Management maintains conversation context. Using state machines or reinforcement learning, the dialog manager ensures coherent and context-aware interactions.
- Text-to-Speech (TTS) converts text responses into speech. This process involves phoneme generation, prosody modeling, and waveform synthesis to produce natural-sounding speech.
{{black-cta}}
Difference Between NLU And NLG In Voice Chatbots
Natural Language Understanding (NLU) and Natural Language Generation (NLG) are key parts of voice chatbots, each with a specific role:
- NLU helps the chatbot understand what you’re saying. It breaks down your speech to figure out your intent and the important details (entity extraction and context understanding).
- NLG is about crafting a response. Once the chatbot understands your input, it uses NLG to create a natural and relevant reply.
Voice Chatbot Use Cases In Different Industries (Examples)
Voice chatbots (or voice assistants) have been incredibly useful across many industries. Here are some examples of how different sectors are using voice chatbots:
Benefits Of Voice Chatbots For Business
A study by Capgemini shows that 77% of consumers who have used voice chatbots report a positive experience, demonstrating their significant potential to enhance customer interactions and streamline operations for businesses. Voice chatbots can:
- Provide 24/7 customer support. This leads to higher customer satisfaction by resolving issues promptly.
- Reduce operational costs by automating routine tasks. This minimizes the need for human agents.
- Increase accessibility for users with disabilities or those preferring voice interactions. This broadens the customer base.
- Boost conversion rates by guiding customers through purchasing decisions. Instant, accurate responses help increase sales.
- Enable scalable customer support. They can handle multiple interactions simultaneously without needing more staff.
How To Implement A Voice Chatbot For Your Business Using OpenAI’s API (Voice Chatbot Setup And Installation Guide in Python)
Follow this guide to build a voice chatbot for your business using OpenAI’s API:
- Set up your environment and make sure you have the tools installed, such as the OpenAI library.
# Install the OpenAI Python client library
pip install openai
- Create an OpenAI account and generate an API key from the dashboard. Then, set up the OpenAI client in your Python script using your key.
import openai
openai.api_key = 'your-api-key-here'
- Use a speech-to-text service to convert voice input to text. We recommend Google Cloud Speech-to-Text, but feel free to use any service of your choice.
from google.cloud import speech_v1p1beta1 as speech
import io
def transcribe_audio(audio_file_path):
client = speech.SpeechClient()
with io.open(audio_file_path, "rb") as audio_file:
content = audio_file.read()
audio = speech.RecognitionAudio(content=content)
config = speech.RecognitionConfig(
encoding=speech.RecognitionConfig.AudioEncoding.LINEAR16,
sample_rate_hertz=16000,
language_code="en-US",
)
response = client.recognize(config=config, audio=audio)
for result in response.results:
return result.alternatives[0].transcript
audio_file_path = "path_to_your_audio_file.wav"
transcribed_text = transcribe_audio(audio_file_path)
- Use OpenAI’s API for NLP and semantic search for querying the knowledge base (KB).
import openai
openai.api_key = 'your-api-key-here'
def generate_response(prompt):
response = openai.Completion.create(
engine="text-davinci-003",
prompt=prompt,
max_tokens=150
)
return response.choices[0].text.strip()
user_query = transcribed_text
response_text = generate_response(user_query)
- Use a Text-to-Speech service to generate audio output from text. We’re using Google Cloud here.
from google.cloud import texttospeech
def synthesize_speech(text, output_file_path):
client = texttospeech.TextToSpeechClient()
input_text = texttospeech.SynthesisInput(text=text)
voice = texttospeech.VoiceSelectionParams(language_code="en-US", ssml_gender=texttospeech.SsmlVoiceGender.NEUTRAL)
audio_config = texttospeech.AudioConfig(audio_encoding=texttospeech.AudioEncoding.MP3)
response = client.synthesize_speech(input=input_text, voice=voice, audio_config=audio_config)
with open(output_file_path, "wb") as out:
out.write(response.audio_content)
synthesize_speech(response_text, "response.mp3")
That’s it! Note that this process is highly technical and requires extensive coding knowledge. If you are looking for an easier, no-code option, read on!
{{blue-cta}}
The Best Voice Chatbot Platform: Voiceflow
Voiceflow stands out as the best voice chatbot platform due to its highly collaborative and extensible nature, making it ideal for cross-functional teams. It offers an intuitive creation experience that integrates seamlessly with existing tech stacks, data sets, and various NLUs or LLMs, providing unparalleled control and flexibility.
Voiceflow’s platform supports advanced orchestration of conversational steps and logic, and its developer toolkit allows for extensive customization. By centralizing AI agent management, facilitating real-time collaboration, and providing full observability and control, Voiceflow ensures teams can rapidly build, deploy, and scale AI agents across multiple use cases.
With Voiceflow, you’re not just building chatbots; you’re crafting sophisticated AI agents that can transform customer experiences and drive business efficiencies. Get started today—it’s free!
{{button}}
Frequently Asked Questions
What are the key features of a voice chatbot?
Voice chatbots come with automatic speech recognition (ASR) to convert spoken language into text and natural language processing (NLP) to understand and respond to user queries. They also offer text-to-speech (TTS) to produce natural-sounding speech, along with intent recognition, dialogue management, and multi-language support.
Can voice chatbots understand different languages?
Yes, voice chatbots can understand different languages. They use advanced natural language processing (NLP) and machine learning models trained on multilingual datasets to understand and respond accurately in multiple languages.
Can voice chatbots be integrated with other systems?
Absolutely, voice chatbots can be integrated with various systems such as CRM, ERP, and other business tools. This integration enables them to fetch and update data, provide personalized responses, and streamline business processes. This can be easily done in Voiceflow.
How do voice chatbots ensure data privacy?
Voice chatbots ensure data privacy by encrypting data during transmission and storage and adhering to compliance standards like GDPR and HIPAA. They also implement user authentication and access controls to prevent unauthorized access to sensitive information.
Voice AI vs. text-based AI: key differences
Unlike traditional chatbots that rely on text input, voice chatbots use advanced speech recognition and natural language processing (NLP) to understand spoken language and respond with human-like speech.
Start building AI Agents
Want to explore how Voiceflow can be a valuable resource for you? Let's talk.
